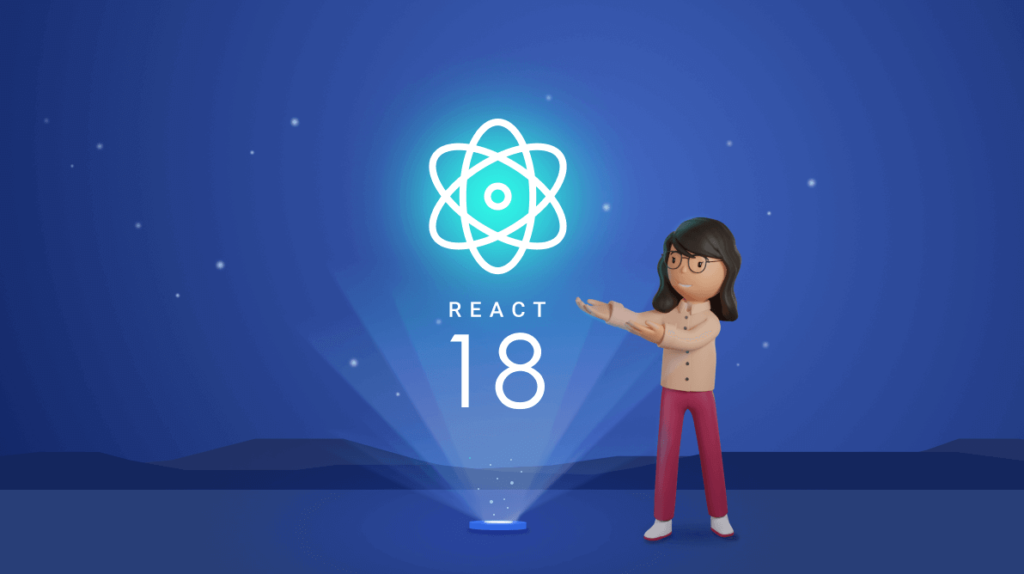
Before we start with new React hooks, let’s first define hooks and why we need them.
A React Hook is a new feature to use React. Component
features such as The state object, Life-cycle events, context, refs etc in a functional component.
Hooks enable us to use “class-features” In React by providing a set of built-in functions such as:
- The
useState()
hook for using states from function components. - The
useEffect()
hook for performing side effects from function components. It’s equivalent to life-cycle methods likecomponentDidMount
,componentDidUpdate
, andcomponentWillUnmount
in React classes. - The
useContext()
hook for subscribing to React Context from a function component. - The
useReducer()
hook for managing the state of components with a reducer. useRef
for React Refs.- The
useCallback
hook for callbacks. - The
useMemo
hook for memoized values. - The
useImperativeMethods
hook for imperative methods. - The
useMutationEffect
hook for mutation effects. - The
useLayoutEffect
hook for layout effects.
we can also create our custom Hooks to re-use any stateful behaviour between different components.
React 18 is a major release that comes with new features, such as concurrent rendering, automated batching, transitions, new APIs and hooks. In this article, we will cover the five new hooks that arrived in React 18:
- useId
- useDeferredValue
- useTransition
- useSyncExternalStore
- useInsertionEffect
useId
Until version 18, React did not offer any good way to generate unique IDs that could be used for server- and client-rendered content. The HTML markup that was rendered on the client should match the one rendered on the server. Otherwise, React server hydration mismatch error will be generated.
UseIdExample.jsx
[React] import { useId } from “react”; const UseIdExample = props => { const uid = useId(); return (useTransition
By default, all React state updates are urgent. Different state updates in our application compete for the same resources, slowing it down. The useTransition React hook solves this problem by letting us mark some state updates as non-urgent. This allows urgent state updates to interrupt those with a lower priority.
[React] import {useState, useTransition} from “react”; function SearchPage() { const [isPending, startTransition] = useTransition(); const [input, setInput] = useState(“”) const [list, setList] = useState([]); const listSize = 30000 function handleChange(e) { setInput(e.target.value); startTransition(() => { const listItems = []; for (let i = 0; i < listSize; i++){ listItems.push(e.target.value); } setList(listItems); }); } return (useDeferredValue
The useDeferredValue hook allows us to defer the re-rendering of a non-urged state update. Like the useTransition hook, the useDeferredValue hook is a concurrency hook. The useDeferredValue hook allows a state to keep its original value while it is in transition.
[React] import { useState, useTransition, useDeferredValue } from “react”; function SearchPage() { const [,startTransition] = useTransition(); const [input, setInput] = useState(“”) const [list, setList] = useState([]); const listSize = 30000 function handleChange(e) { setInput(e.target.value); startTransition(() => { const listItems = []; for (let i = 0; i < listSize; i++){ listItems.push(e.target.value); } setList(listItems); }); } const deferredValue = useDeferredValue(input); return (useSyncExternalStore
It allows our React application to subscribe to and read data from external libraries. The useSyncExternalStore hook uses the following declaration:
const state = useSyncExternalStore(subscribe, getSnapshot[, getServerSnapshot]);
This signature contains the following:
- state: the value of the data store that the useSyncExternalStore hook returns.
- subscribe: registers a callback when the data store changes.
- getSnapshot: returns the data store current value.
- getServerSnapshot: returns the snapshot used during server rendering.
useInsertionEffect
The useInsertionEffect hook is another new React hook that works with libraries. However, the useInsertionEffect hook works with CSS-in-JS libraries instead of data stores. This hook addresses style rendering performance issues. It styles the DOM before reading the layout in the useLayoutEffect hook.
Please check here if you want to know more about React 18 new hooks.