Redis is a popular in-memory data store widely used for caching, real-time analytics, message brokering, and other use cases. It supports various data structures such as strings, hashes, lists, sets, and sorted sets with range queries, bitmaps, hyperloglogs, and geospatial indexes. It can be used as a primary data store or as a cache to speed up access to data stored in a traditional persistent database. Redis is commonly used in web applications to store session information and other types of temporary data.
In summary, Redis is a fast and reliable in-memory data store that can be used as a database, cache, or message broker. Its support for transactions and ability to scale make it a popular choice for web applications and other types of distributed systems
How it works.
When we deploy the Redis instance for the first time, In the initial stage Redis doesn’t have the data inside of it aside from its configuration data combined with default settings. We can populate data in a few ways.
One of the ways is when the web server makes a first request for the first piece of data to Redis and it does not have the data it is called a cache miss, then the web server directly requests the database for the data. On successful retrieval of data from the database it saves to the Redis cache. When in the future any web server retrieves the same data it is called a cache hit.
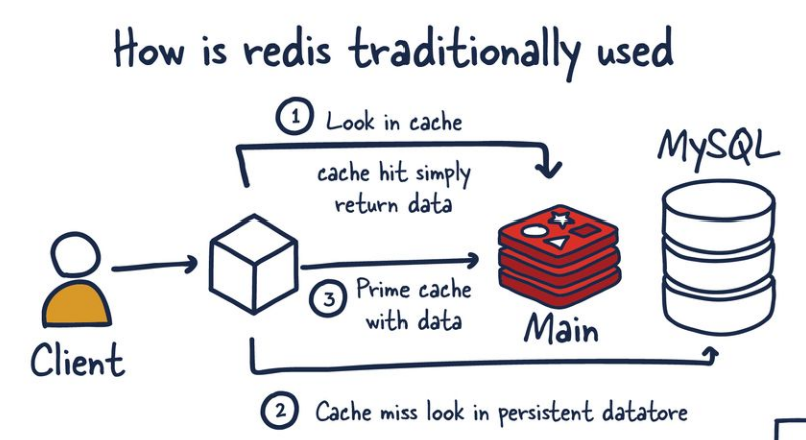
Another way is the use of a cache worker. It will work like a bridge between the Redis cache and the database. In other words, the web server doesn’t want to agonize about the first cache. The cache worker monitors the database for any changes to actual data and when it finds any change it populates the data to the Redis cache automatically.
How it helps me!
I was developing E- shop app in which I used a product recommendation system that shows the products on the base of their recent history and last viewed, But the problem I was facing is Lambda time-out.
Lambda Timeout is the maximum amount of time in seconds that a Lambda function can run.
All my APIs run on AWS Lambda which is set to the default time of 30sec. So, whenever my Layout page tries to get data from the recommendation system, the layout has to stay for the response till the recommendation API response But it takes more than the default time, and layouts Lambda time-out and shows an error.
The main reason for my problem is that the recommendation API has to process data every time that’s why it takes time to respond. On another hand, I didn’t want that my recommendation data change frequently on layout despite of that we use Redis to store my data for some time until there will be another request to the recommendation API. So, that’s why I thought that we can cache our data.
How to implement Redis.
In Redis as per my use case, I use only get, set, and expire time functions.
SET — Set key
to hold the string value
. If key
already holds a value, it is overwritten, regardless of its type. Any previous time to live associated with the key is discarded on the successful SET
operation.
if redis.get("recommanded_response") == None: response = requests.get(url, headers=headers) print(response.json()["recommended"][0]) redis.set( "recommanded_response", json.dumps(response.json()["recommended"][0]), ex=300 ) else: print(redis.get("recommanded_response"))
Get — Return the old string stored at key, or nil if the key did not exist. An error is returned and SET
aborted if the value stored at the key is not a string.