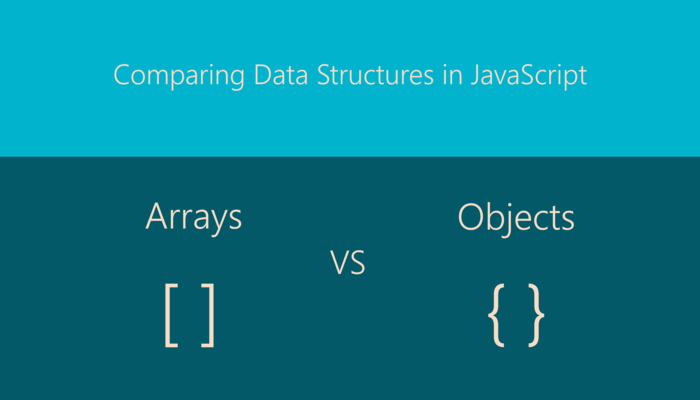
Introduction
JavaScript is a versatile language that is used for developing web applications. It has a number of data structures that can be used to store and manipulate data. Two of the most commonly used data structures in JavaScript are arrays and objects.
Arrays and objects are both used to store data, but they have some fundamental differences. In this article, we will discuss the differences between JavaScript arrays and objects and which is better in certain situations.
Arrays
In JavaScript, an array is a data structure that is used to store a collection of values. The values can be of any data type, including strings, numbers, objects, and even other arrays. An array is created by enclosing a list of values inside square brackets [ ] and separating each value with a comma. Here is an example:

Arrays in JavaScript are zero-indexed, which means that the first element of an array has an index of 0. We can access the values of an array using its index. Here is an example:

Arrays in JavaScript are mutable, which means that we can add or remove elements from them. Here are some methods that can be used to manipulate arrays:
- push() — adds an element to the end of an array
- pop() — removes the last element from an array
- shift() — removes the first element from an array
- unshift() — adds an element to the beginning of an array
- splice() — adds or removes elements from an array at a specified position
Objects
An object in JavaScript is a data structure that is used to store a collection of key-value pairs. The keys in an object are strings, and the values can be of any data type, including strings, numbers, objects, and even other arrays. An object is created by enclosing a list of key-value pairs inside curly braces { } and separating each pair with a comma. Here is an example:

We can access the values of an object using its keys. Here is an example:

Objects in JavaScript are also mutable, which means that we can add or remove key-value pairs from them. Here are some methods that can be used to manipulate objects:
- Object.assign() — copies the values of all enumerable properties from one or more source objects to a target object
- Object.keys() — returns an array of the keys in an object
- Object.values() — returns an array of the values in an object
- delete — removes a property from an object
Differences between Arrays and Objects
Now that we know what arrays and objects are, let’s discuss the differences between them.
- Ordering: Arrays are ordered, which means that the elements are arranged in a specific sequence, and each element has a unique index. Objects, on the other hand, are unordered, and their elements are stored as key-value pairs, which means that each element has a key and a corresponding value.
- Accessing Data: In an array, we can access the data using its index, which is an integer that represents its position in the array. In contrast, objects allow us to access the data using its key, which is a string that represents the name of the element.
- Data Types: Arrays can store any data type, including strings, numbers, objects, and even other arrays. In contrast, objects can store any data type for the value, but the keys must be strings.
- Mutability: Both arrays and objects are mutable, which means that we can add or remove elements from them. However, when an element is removed from an array, the remaining elements shift to fill the gap, and the indexes are updated accordingly. In contrast, when an element is removed from an object, the remaining elements do not shift.
- Length: Arrays have a length property that returns the number of elements in the array. In contrast, objects do not have a length property.
- Performance: Arrays are generally faster than objects when it comes to accessing data because they use integer indexes, which can be optimized by the browser. In contrast, objects use strings as keys, which are not as efficient for optimization.
In summary, arrays are best used when we need to store data in a specific order and manipulate it based on its position in the collection. Objects are best used when we need to store data as key-value pairs and access it based on its key. Choosing the right data structure for the task at hand can make our code more efficient and easier to maintain.
Conclusion
In conclusion, both arrays and objects are useful data structures in JavaScript, but they have different purposes and functionalities. Arrays are best used when we need to store a collection of data in a specific order and manipulate it based on its position in the collection. On the other hand, objects are best used when we need to store a collection of data that has key-value pairs and we need to access the data based on its key.
It’s important to choose the right data structure for the task at hand, as using the wrong one can result in inefficient code or make it difficult to achieve the desired outcome. In some cases, it might even be necessary to use both arrays and objects together to accomplish a specific task.