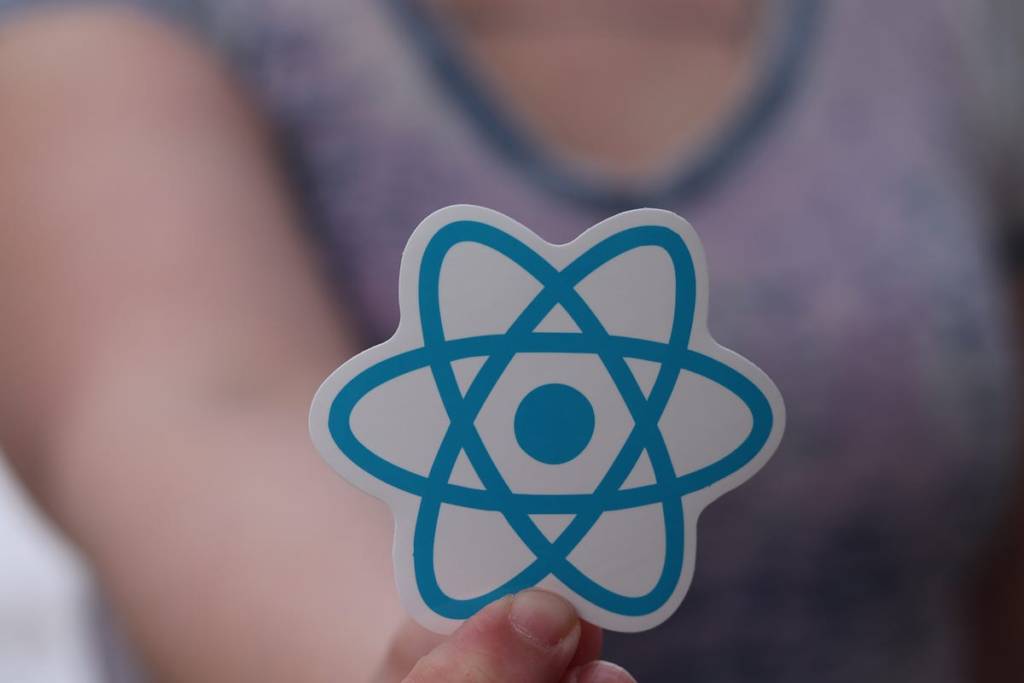
What are hooks in react?
Hooks are the most powerful feature of React, They enable React functional components to use React features that were previously only available in React class components.
In simpler words, they are functions that bring the power of React class components to functional components, giving you a cleaner way to combine them.
React comes with some built-in hooks, the most commonly used ones being useState, useRef, and useEffect, you can deep dive into hooks in the official documentation: https://reactjs.org/docs/hooks-intro.html.
What are custom hooks and their uses?
According to react documentation,
“A custom Hook is a JavaScript function whose name starts with ”use” and that may call other Hooks.”
If you have code in a component that you think would make sense to extract, reuse elsewhere or to keep the component simpler, you can take that out into a function.
Let’s learn this through an example :
We will create a custom hook that fetches that fetches TODO list from an API.
import { useEffect, useState } from "react"; export function useFetch(url) { const [data, setData] = useState(null); const [isLoading, setIsLoading] = useState(false); const [error, setError] = useState(false); const fetchData = async () => { setIsLoading(true); try { const result = await fetch(url); const data = await res.json(); setData(data); } catch (error) { setError(error); } finally { setIsLoading(false); } }; useEffect(() => { fetchData(); }, []); return {data,isLoading,error} }
Now to use this custom hook you just need to import this where you want to fetch data from your link like this
:const {data,isLoading, error} = useFetch(// here you will pass url of the API)
Some of the rules you must keep in mind while working with customs hooks
- You should always use Hooks at the top level of your React component.
- Call Hooks from React function components or call Hooks from custom Hooks.
- Never call Hooks inside loops, conditions, or nested functions.
For more, you can check the official react documentation for custom hooks: https://reactjs.org/docs/hooks-custom.html