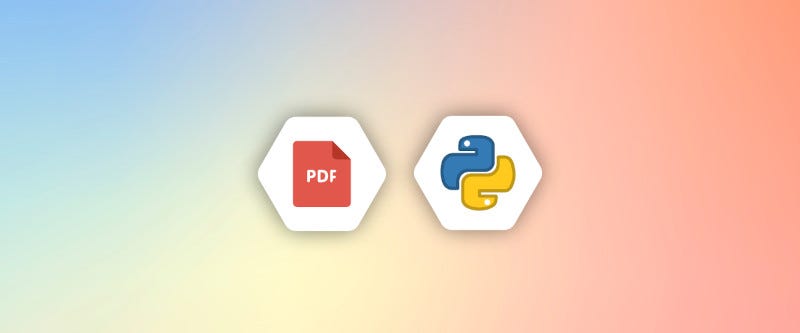
Do you need to generate a PDF file from a html string or a text document? If so, Python’s pdfkit library can help you with that! In this article, we’ll go over how to use pdfkit to generate PDFs, with a step-by-step example to help illustrate the process.
Step 1: To use pdfkit, you’ll need to install it. You can do this by running the following command in your terminal or command prompt:
[Python] pip install pdfkit [Python]Step 2: Import the Library Once you have pdfkit installed, you’ll need to import it into your Python script. To do this, add the following line of code at the top of your script:
[Python] import pdfkit [Python]Step 3: Generate PDF from a html String The simplest way to generate a PDF with pdfkit is to convert a string into a PDF. Here’s an example:
[Python] html_string = ‘Hello World!
This is a test PDF generated from a string.
‘ pdfkit.from_string(html_string, ‘hello.pdf’) [Python]In this example, html_string
is a string containing some HTML code. The pdfkit.from_string
function converts this HTML code into a PDF file named “hello.pdf”.
Step 4: Generate PDF from a Text Document You can also use pdfkit to generate a PDF from a text document. For example, here’s how you could generate a PDF from a text file named “example.txt”:
[Python] pdfkit.from_file(‘example.txt’, ‘example.pdf’) [Python]Step 5: Customizing the PDF Output You can customize the PDF output by specifying options as a dictionary. For example, you can specify the page size, margins, and other options. Here’s an example:
[Python] options = { ‘page-size’: ‘Letter’, ‘margin-top’: ‘0mm’, ‘margin-right’: ‘0mm’, ‘margin-bottom’: ‘0mm’, ‘margin-left’: ‘0mm’, ‘encoding’: “UTF-8”, ‘no-outline’: None } pdfkit.from_file(‘example.txt’, ‘example_custom.pdf’, options=options) [Python]Conclusion
In conclusion, generating PDFs with Python’s pdfkit library is a straightforward process. By following the steps outlined in this article, you should be able to easily generate PDFs from html strings and text documents. For more detailed explanation about pdfkit read it’s documentation here.