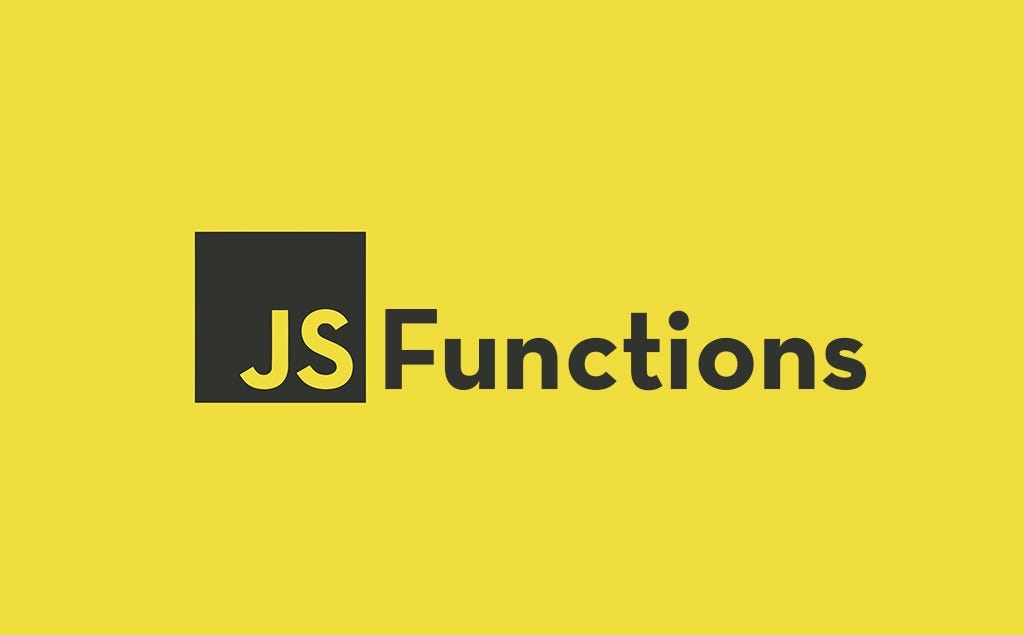
What is Function in JavaScript?
In JavaScript, a function is a block of code that can be executed when it is called, and it can also receive input (known as “arguments”) and return output (known as “return value”). Functions are a fundamental part of the JavaScript language and are used to organize and modularize code.
A function that does not take an argument and doesn’t return anything.
function sayHelloWorld() {
console.log('Hello World!')
}
sayHelloWorld(); // logs "Hello World!"
A function that does take an argument and doesn’t return anything.
function congratulation(name) {
console.log(`Congratulations, ${name}! 🎉🎉`)
}
congratulation("Harshit Goyal"); // logs "Congratulations, Harshit Goyal! 🎉🎉"
here is an example, congratulation is a function that takes a name as an argument and returns undefined whose work is to console the congratulations message.
A function that does not take an argument and returns a value.
function getRandomNumber() {
return Math.floor(Math.random() * 100);
}
const randomNumber = getRandomNumber();
console.log(randomNumber); // Output: a random number between 0 and 99
In this example, getRandomNumber
is a function that does not take any arguments but returns a random integer between 0 and 99 using the Math.random()
and Math.floor()
methods. We then assign the returned value to the variable randomNumber
and log it to the console.
Note that even though this function doesn’t take any arguments, it can still access variables outside its scope. For example, it could use a global variable or a variable declared in an outer function.
A function that takes a parameter and returns a value.
function square(number) {
return number * number;
}
const value = square(10);
console.log(value); // logs 100
Types of functions in javascript
There are several types of functions in JavaScript, including:
- Function Declarations: These are the most common type of functions in JavaScript. They are defined using the function keyword followed by the function name, a set of parentheses for any parameters, and a block of code between curly braces.
- Function Expressions: These are similar to function declarations, but they are assigned to a variable. Function expressions are created using the var or let keyword and the function keyword.
- Arrow Functions: Arrow functions were introduced in ECMAScript 6 (ES6) and are a more concise way of defining functions. They use the => operator instead of the function keyword and do not have their own this binding.
- IIFE (Immediately-Invoked Function Expressions): An IIFE is a function that is immediately invoked after it is defined. This pattern is used to create a scope and execute a function immediately after it is defined.
- Constructor Functions: These are functions that are used to create objects. When a constructor function is called with the new keyword, a new object is created and the constructor function’s code is executed.
- Generator Functions: These are functions that can be paused and resumed multiple times, allowing them to produce a series of values over time. They are defined using the function* keyword.
- Async Functions: These are functions that enable asynchronous programming, allowing you to write asynchronous code that looks like synchronous code. They are defined using the async keyword and return a promise.
Each of these types of functions has its own specific use case, and it’s essential to understand their behavior and how to use them correctly.
// function declaration
function greet(name) {
console.log("Hello, " + name + "!");
}
// function expression
const greet = function(name) {
console.log("Hello, " + name + "!");
};
// arrow function
const greet = (name) => {
console.log("Hello, " + name + "!");
};
// concise arrow function
const greet = name => console.log("Hello, " + name + "!");
// Immediately-Invoked Function Expressions
(() => {
console.log("I'm IIFE")
})()
Functions are a powerful and flexible feature of JavaScript, and they are used to organize and structure code, create reusable code snippets, and perform actions in response to events.
Where can IIFE be used?
It can be used in scenarios where you need to run the function only once, like fetching some initial data, setting some configuration values, checking system status on startup, etc.
Declarative v/s Imperative
In JavaScript, “declarative” and “imperative” are two different programming styles that are used to achieve the same goal.
“Declarative” programming is a style where the focus is on what the program should accomplish, rather than how it should accomplish it. Declarative code expresses the logic of a computation without describing its control flow. This style is often associated with functional programming and is characterized by code that is more readable, easier to understand, and less prone to bugs.
Here’s an example of declarative code that filters and maps an array:
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(n => n % 2 === 0).map(n => n * 2);
On the other hand, “Imperative” programming is a style where the focus is on how the program should accomplish a task, rather than what it should accomplish. Imperative code describes the specific steps that the program should take in order to complete a task. This style is often associated with object-oriented programming and is characterized by code that is more complex and harder to understand.
Here’s an example of imperative code that filters and maps an array:
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = [];
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 === 0) {
evenNumbers.push(numbers[i] * 2);
}
}
Both styles can be used to accomplish the same task, but declarative programming is generally considered to be more readable, maintainable, and easier to reason about. It encourages to use of higher-order functions and composes them to express computation.
Imperative programming is considered to be more low-level, and it usually requires more lines of code and more explicit control flow statements. It’s often used when you need more fine-grained control over the execution flow of your code.
Functions are first-class objects
Functions can also be assigned to variables and passed as arguments to other functions, which is known as the “first-class functions” concept in javascript.
Anonymous Functions
Functions can be created without a name and assigned to a variable.
const myFunc = function() {
console.log("Hello, World!");
};
myFunc(); // Output: "Hello, World!"
Higher-Order Functions
Functions that take other functions as arguments or return a function are called higher-order functions.
function multiplyBy(factor) {
return function(number) {
return number * factor;
}
}
const double = multiplyBy(2);
console.log(double(5)); // Output: 10
In this example, multiplyBy
is a higher-order function that returns a function that multiplies its argument by the factor
passed to multiplyBy
.
Closures
A closure is created when a function returns another function and the inner function has access to the outer function’s variables and parameters. Closures are often used in JavaScript for data hiding and encapsulation.
function createCounter() {
let count = 0;
return function() {
count++;
console.log(count);
}
}
const counter = createCounter();
counter(); // Output: 1
counter(); // Output: 2
counter(); // Output: 3
In this example, createCounter
returns a function that has access to the count
variable declared in the outer function. The returned function increments the count
variable each time it is called and logs the current count to the console.
These are some important concepts related to functions in JavaScript. Functions are a powerful tool in JavaScript that enable a wide range of programming techniques and patterns.
Hope this article is helpful for you and you must have learned Functions in JavaScript.
Big thanks for your precious time & please share your feedback.
Happy Coding!! Keep Learning & Keep Growing.