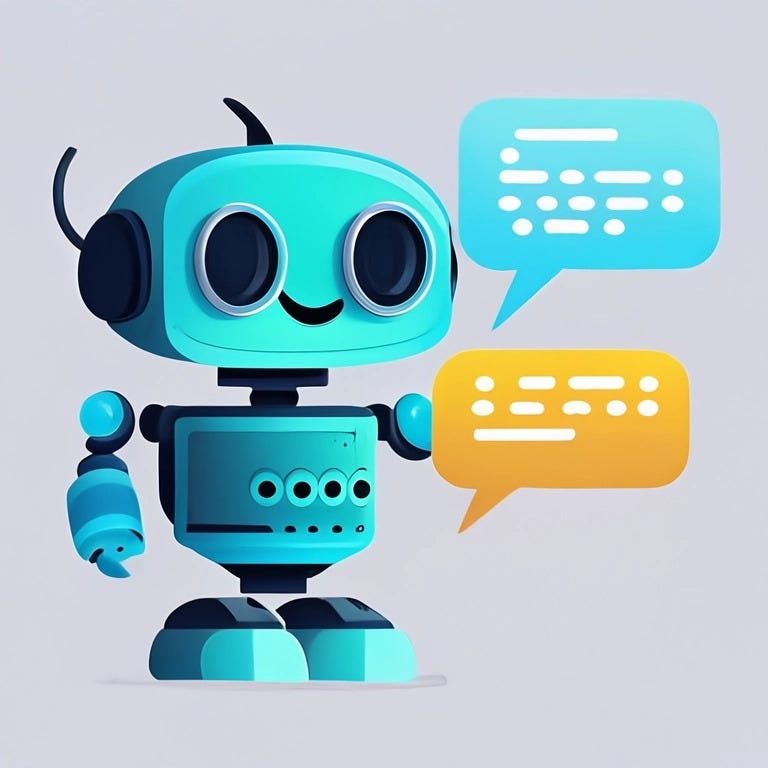
Chatbots have become an increasingly popular way for businesses to automate customer service and improve user engagement. One of the most powerful tools available for developing chatbots is the ChatGPT API, an AI-powered language model trained by OpenAI.
In this blog post, we’ll explore how to interact with the ChatGPT API in Python and how to use it to build a powerful chatbot.
Getting started with the OpenAI API
Before we can start using the ChatGPT API in Python, we’ll need to set up an account and obtain an API key. Once we have an API key, we can use it to make requests to the API and generate responses to user queries.
To start, we’ll need to install the openai
Python library using pip. We can do this by running the following command in our terminal:
Once we have installed the openai
library, we can import it into our Python code and initialize an instance of the openai.Completion
class, which will handle the communication with the ChatGPT API. Here’s an example of how we can do this:
Here, we’re importing the openai
library and the os
library, which we’ll use to store our API key as an environment variable. We’re then setting the api_key
property of the openai
module to our API key, which we’ve stored in an environment variable called OPENAI_API_KEY
. Finally, we’re initializing an instance of the openai.Completion
class and storing it in a variable called completion
.
Generating responses with the ChatGPT API
Now that we have initialized an instance of the openai.Completion
class, we can use it to generate responses to user queries. To do this, we’ll need to provide a prompt to the API, which provides context for the user’s query and helps the ChatGPT model generate a relevant response.
Here’s an example of how we can generate a response to a user query using the ChatGPT API:
[Python] # Define prompt prompt = “Hello, how can I help you today?” [Python]In this first step, we’re defining a prompt that the user will see when they interact with our chatbot. This prompt asks the user how we can help them.
[Python] # Generate response response = completion.create( engine=”davinci”, prompt=prompt, max_tokens=60, temperature=0.5, n=1, stop=None, timeout=15, )[“choices”][0][“text”] # Print response print(response) [Python]In this step, we’re using the create
method of the completion
object to generate a response to the prompt. We’re passing several arguments to the create
method to control the behavior of the API:
engine
: This argument specifies which language model to use. In this case, we’re using thedavinci
engine, which is the most advanced engine available for the ChatGPT API.prompt
: This argument specifies the prompt that the user sees.max_tokens
: This argument limits the length of the response to 60 tokens. A token is a unit of text, typically a word or a punctuation mark.temperature
: This argument controls the creativity of the response. A higher temperature results in more creative responses, while a lower temperature results in more predictable responses.n
: This argument specifies how many responses to generate. In this case, we’re generating only one response.stop
: This argument specifies a list of strings that the response should not contain. We’re setting this argument toNone
, which means that the response can contain any string.timeout
: This argument specifies the maximum amount of time that the API should take to generate a response. In this case, we’re setting it to 15 seconds.
Once we’ve generated the response, we’re storing it in a variable called response
. Finally, we’re printing the response to the console so that the user can see it.
Let’s continue with our example and add some more code to create a basic chatbot.
[Python] import openai import time # Set up the OpenAI API key openai.api_key = “YOUR_API_KEY_HERE” # Set up the ChatGPT API parameters engine = “davinci” # The AI model to use max_tokens = 150 # The maximum number of tokens to generate per request temperature = 0.5 # Controls the “creativity” of the generated text n = 1 # The number of responses to generate per request stop = “\n” # The stop sequence for the API # Initialize a dialogue history list dialogue_history = [] # Define a function to generate responses def generate_response(prompt): # Add the user’s prompt to the dialogue history dialogue_history.append(“You: ” + prompt) # Concatenate the dialogue history into a single string dialogue_string = “\n”.join(dialogue_history[-3:]) # Call the ChatGPT API to generate a response response = openai.Completion.create( engine=engine, prompt=dialogue_string, max_tokens=max_tokens, temperature=temperature, n=n, stop=stop ).choices[0].text.strip() # Add the bot’s response to the dialogue history dialogue_history.append(“Bot: ” + response) # Return the bot’s response return response # Define a function to handle user input def handle_input(): # Get user input user_input = input(“You: “) # Generate a response response = generate_response(user_input) # Print the bot’s response print(“Bot: ” + response) # Pause for a moment to avoid hitting the ChatGPT API rate limit time.sleep(1) # Main loop while True: # Handle user input handle_input() [Python]Here’s what this code does:
- It sets up the OpenAI API key and the ChatGPT API parameters, just like in the previous example.
- It initializes a
dialogue_history
list to keep track of previous user inputs and responses. - It defines a
generate_response
function that takes a user prompt as input, adds the prompt to the dialogue history, calls the ChatGPT API to generate a response based on the dialogue history, adds the response to the dialogue history, and returns the response. - It defines a
handle_input
function that prompts the user for input, generates a response using thegenerate_response
function, prints the response to the console, and pauses for a moment to avoid hitting the ChatGPT API rate limit. - It sets up a main loop that repeatedly calls the
handle_input
function to handle user input and generate responses.
Here’s an example conversation that you could have with the chatbot using the code
[VB.NET] You: Hi there, how are you doing today? Bot: I’m doing well, thanks for asking. How can I assist you? You: Can you recommend a good Italian restaurant in Delhi? Bot: Of course! There are several great Italian restaurants in Delhi. Some popular options include Diva Italian, Tonino, and Artusi Ristorante. Which one would you like more information about? You: Tell me more about Artusi Ristorante. Bot: Artusi Ristorante is a popular Italian restaurant located in Greater Kailash. They specialize in traditional Italian cuisine, with an emphasis on fresh ingredients and homemade pastas. The restaurant has a cozy, elegant atmosphere and an extensive wine list. Would you like more information on their location or menu? You: What are some of their popular dishes? Bot: Some popular dishes at Artusi Ristorante include their ravioli al tartufo (ravioli stuffed with truffle cream sauce), spaghetti alla carbonara (spaghetti with egg, bacon, and pecorino cheese), and panna cotta (a traditional Italian dessert made with cream and fruit). They also have a great selection of wines to pair with your meal. You: That all sounds delicious! Do they take reservations? Bot: Yes, Artusi Ristorante does take reservations. You can call them at +91 11 49066666 to make a reservation, or you can book a table on their website. You: Great, thanks for the recommendation. Do you have any suggestions for things to do in Delhi besides restaurants? Bot: Absolutely! There are plenty of things to see and do in Delhi. Some popular attractions include the Red Fort, Humayun’s Tomb, and the Lotus Temple. There are also several great museums and galleries, such as the National Museum and the National Gallery of Modern Art. Do you have any particular interests or preferences? You: I’m interested in history and culture. Bot: In that case, I would definitely recommend visiting the Qutub Minar and the Jama Masjid. Both are historic landmarks with beautiful architecture and fascinating stories. You might also enjoy exploring the Chandni Chowk market, which is one of the oldest and busiest markets in Delhi. It’s a great place to experience the local culture and try some delicious street food. You: That all sounds wonderful! Thank you for your help. Bot: You’re welcome! Enjoy your time in Delhi. [VB.NET]Overall, Chat GPT API offers a powerful and flexible tool for building chatbots that can interact with users in natural language. With its sophisticated natural language processing capabilities, it’s well-suited for a wide range of applications, from customer service to personal assistants.
By following the best practices we’ve outlined and experimenting with different techniques, you can create a chatbot that delivers a smooth, engaging experience for your users. Whether you’re building a simple bot for personal use or developing a more complex application for your business, Chat GPT API is an invaluable resource for building smarter, more effective chatbots.