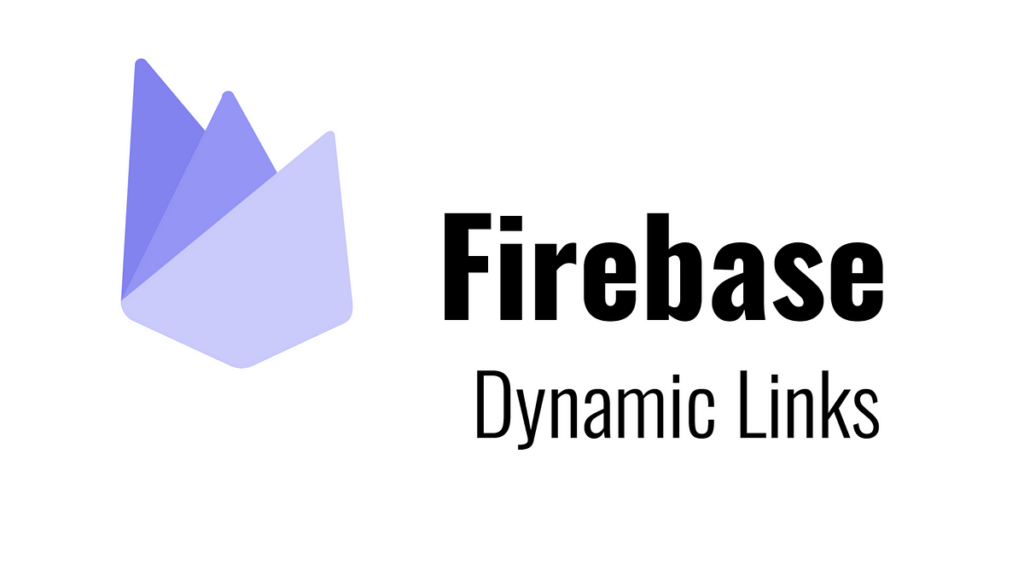
Deep linking improves user engagement and provides a better user experience
In today’s era where everything is connected and we share links more often than before. We also want our customers to reach the desired page, as quickly as possible regardless of the platform they are using.
Deep linking does the work here by providing a clickable link that opens up our app in a smart way to navigate to the desired page.
What is Deep linking?
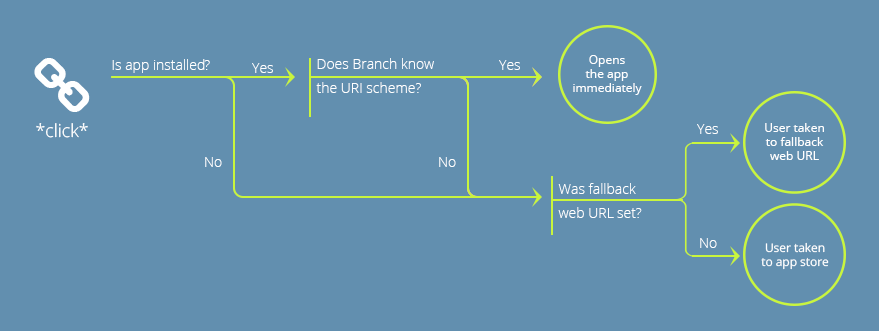
Deep linking is a process that lets us use a single URL to forward our users to a specific location inside an app. It is a technique that allows an app to be opened to a specific UI or resource, in response to some external event.
Steps to Achieve Deep Linking –
Firebase Dynamic Links provides a rich feature for handling deep linking for applications and websites. The best part is that Firebase deep linking is free, for any scale.
Firebase Dynamic Links handles the following scenarios:
- When a user opens Dynamic Links, if our app isn’t installed, the user is redirected(through a fallback URL) to the Play Store or App Store to install our app.
- If the app is installed and the app is not open in the background, then the app is opened and user is redirected to desired screen (registered listener).
- If the app is in the background, then the user is directly navigated to desired screen (registered listener).
We will be following these steps for creating and receiving Dynamic Links –
1. Set up URL Prefix in Firebase and the Dynamic Links SDK in our App
ENABLE FIREBASE DYNAMIC LINKS FOR YOUR FIREBASE PROJECT IN THE FIREBASE CONSOLE.
- Open the Firebase console and select the Firebase project in which you want to add deep linking.
- Create an Android and IOS app in the Firebase project.
- Open Dynamic Links from the side drawer’s Grow section.

- Click on the Get Started button, and create a domain for the link.
- Then, create a URL prefix. A URL prefix is a domain name that uses dynamic links.
- Create a tab, and add the custom, unique domain name of your app. For example, test.page.link. Here, the domain name is postfixed with
page.link
. - Then, follow: Configure → Verify → finish steps.
Install the package to use the dynamic link in our App-
npm i @react-native-firebase/dynamic-links
2. Create Dynamic Links
WE CAN CREATE DYNAMIC LINKS PROGRAMMATICALLY OR BY USING THE FIREBASE CONSOLE.
Dynamic Links programmatically:
To help you here for creating a deep link I would suggest we should keep in mind one question i.e. how we will be reading that link once someone enters our app using that link?
So to answer that we need to make our URI which includes params like — the navigation field to let us know the screen where we will be navigating to and then what will be required parameters for the API that we will be hitting to get data on that screen. (ex. https://example.page.link/navigateTo=ProductsPage&prodid=013PVN )//here we are creating a link to show a product’s detail on the product page
const Link = async (navigateTo: string, productId: string) => { const link = await dynamicLinks().buildShortLink( { link: `${UriPrefix}/app/?navigateTo=${navigateTo}&&productId=${uuid}`, domainUriPrefix: UriPrefix, android: { packageName: androidAppBundleId, //The package name of the Android app in firebase console . fallbackUrl: FallbackUrl, //Sets the link to open when the app isn't installed . }, ios: { bundleId: iosAppBundleId, //The bundle ID of the iOS app in firebase console . appStoreId: AppStoreId, //Sets the link to open when the app isn't installed . }, }, dynamicLinks.ShortLinkType.UNGUESSABLE //Shorten the path to an unguessable string (encodes link) ); return link; };
To extract the App bundleId/packageName from our project code for the device we can use this package — npm i react-native-device-info
import DeviceInfo from 'react-native-device-info'; const AppBundleId= DeviceInfo.getBundleId();
To share the Deeplink we can use this package — npm i react-native-share
3. Handle Dynamic Links in your app
When your app opens, use the Dynamic Links SDK to check if a Dynamic Link was passed to it. If so, get the deep link from the Dynamic Link data and handle the deep link as necessary.
To teach our App how to use Link to navigate to the screen which is intended to be opened when a specific URI is clicked, we should keep our link handling code in a screen which renders first, here am keeping my handler code on ‘Home.tsx’ file.
/** *handle the navigation for different flows from Deeplink * @param url:contains the params to pass while navigation */ const handleDynamicLink = (url: string) => { const params = getParamsFromURL(url); //A utility function that returns a set value pair for the param list of given url const navigateTo = params?.get("navigateTo"); const productId = params?.get("productId") || ""; if (navigateTo) { switch (navigateTo) { case "productPage": navigation.navigate(APP_ROUTES.PRODUCTS_PAGE_ROUTE, { productId }); //navigation to products page with product id which will be used in API to get the product break; default: break; } } }; //executes when user joins with deepLink useEffect(() => { const unsubscribe = dynamicLinks().onLink((link) => { if (link?.url) { handleDynamicLink(link.url); } }); //executes when app is not in background dynamicLinks() .getInitialLink() .then((link) => { if (link?.url) { handleDynamicLink(link.url); } }); // When the component is unmounted, remove the listener return () => unsubscribe(); // eslint-disable-next-line react-hooks/exhaustive-deps }, []);
And That’s It … we are good to share/read deep links for our App.
I hope it is helpful for you to set up a basic Deep Link flow for your project.