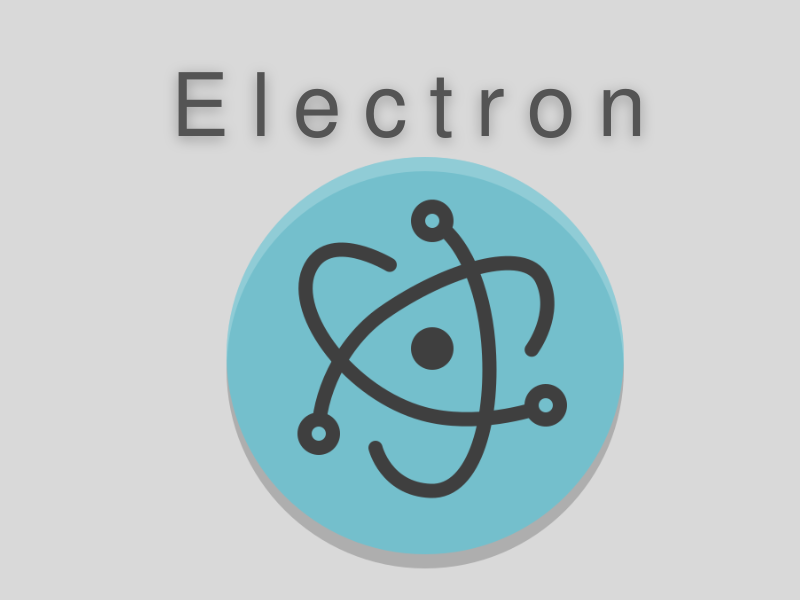
Desktop applications are stand-alone applications that run on a system, they may or may not require Internet access. Examples of such apps are
- Adobe Photoshop
- Microsoft Office applications
- Skype
- Discord
- Slack
There are various tools that can be used to build a desktop app, such as Electron, Winforms, WFP, Swing, JavaFX, etc. In this article, we’ll be getting a tour of Electron JS😀.
Introduction to Electron JS
Electron is a framework that is used to build cross-platform desktop applications using HTML, CSS, and JavaScript. it is an open-source and free project. Electron does not require native development skills for creating apps that run on Windows, macOS, and Linux; By embedding chromium and node.js, it maintains a codebase of JavaScript. Applications that use Electron JS for its development are WhatsApp, Slack, VS code, WordPress, etc.
Build an Electron App
Open the folder in which we want to create the app and initialize an npm package.
The entry file would be main.js
npm init
Now, the next step would be to install an electron. For this, the following command needs to be executed.
npm install electron --save-dev
Create the files index.html and main.js
This is my first Electron App
In the main.js file, import the necessary modules
const { app, BrowserWindow } = require(‘electron’)
Create a function, create a window (), which will contain the property of the new window to be created, and load the html file.
const createWindow = () => { const win = new BrowserWindow({ width: 800, height: 600, webPreferences: { nodeIntegration: true, }, }); win.loadFile("index.html"); };
The above function will be executed when our app is ready,
app.whenReady().then(create window)
To execute, add electron .
to the start
command in the scripts field of package.json. Through this command, the Electron executable will look for the main script in the current directory and run it in dev mode.
Now, Run the command
npm run start
🥳Hurray!
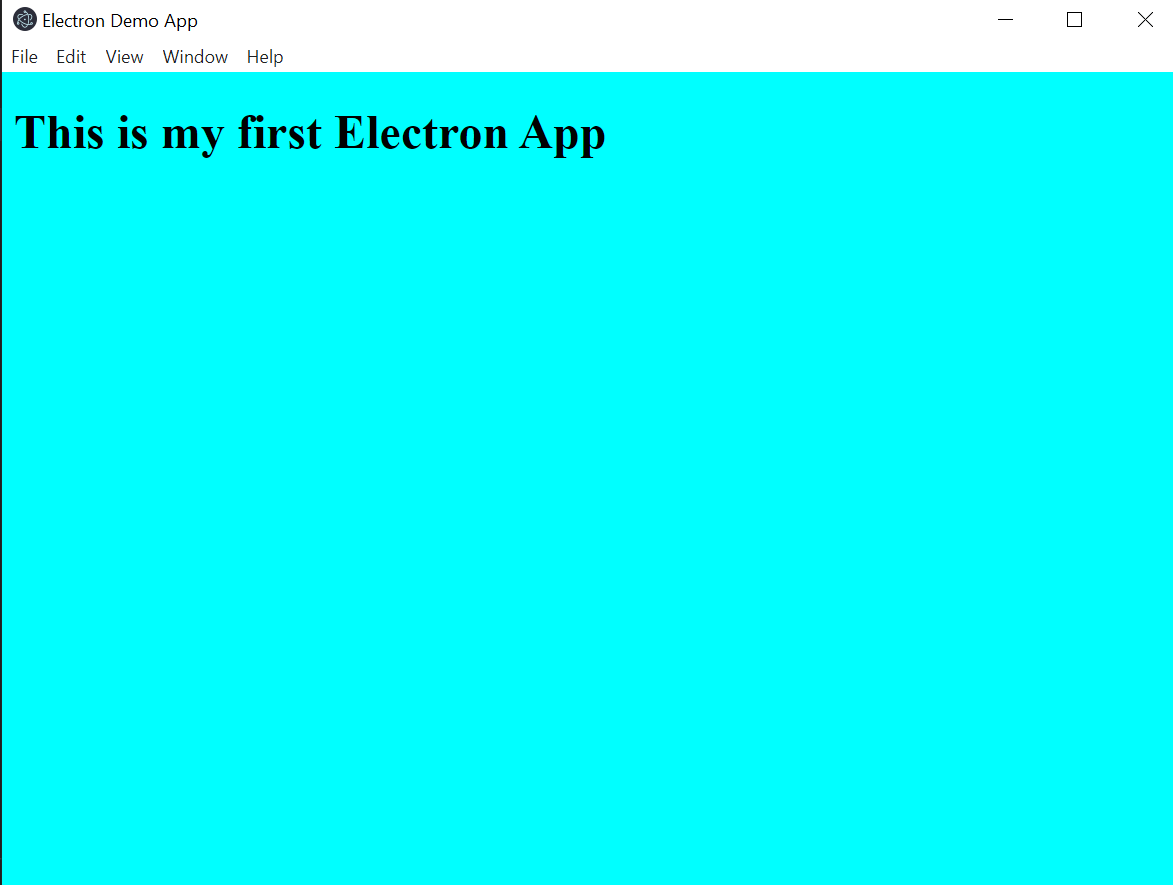
BrowserWindow
The BrowserWindow
class that we have used in the above example helps to modify the look and behavior of the app’s windows by using various options which are optional, few of them are as follows:
- width (Integer): defines the window’s width (pixels). [Default value: 800].
- height (Integer): defines the window’s height (pixels). [Default value: 600].
- useContentSize (boolean): width and height are used as the size of the web page. That is, the actual window size includes the size of the window frame and is slightly larger. [Default value: false]
- center (boolean): shows a window in the center of the screen. [Default value: false]
- minWidth (Integer): defines the window’s minimum width. [Default value: 0]
- minHeight (Integer): defines the window’s minimum height. [Default value: 0]
- maxWidth (Integer): defines the window’s maximum width. [Default value: no limit]
- maxHeight (Integer): defines window’s maximum height. [Default value: no limit]
- resizable (boolean): describes whether window is resizable. [Default value: true]
- movable (boolean) macOS Windows: describes whether window is movable. [Default value: true]
- minimizable (boolean) macOS Windows: describes whether window is minimizable. [Default value: true]
- maximizable (boolean) macOS Windows: describes whether window is maximizable. [Default value: true]
Reference
Electron JS documentation : https://www.electronjs.org/docs/latest/
Thank You
Keep Learning🤓