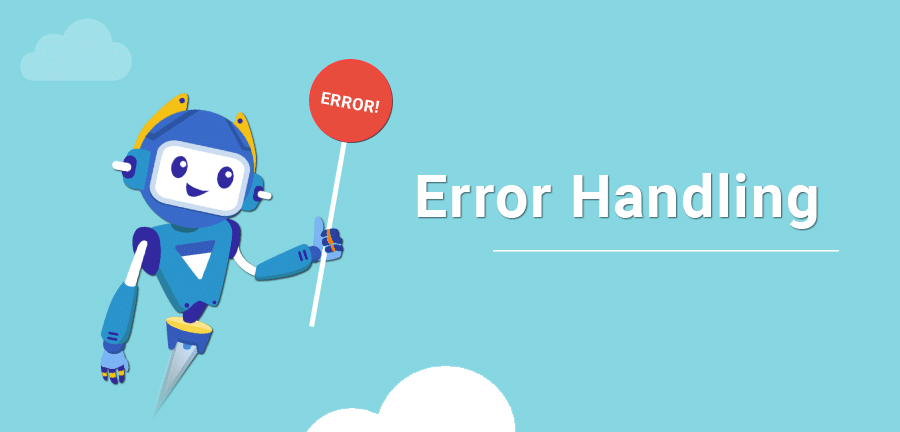
Errors are bound to happen in our applications, whether they’re server-related errors, edge cases, api calling issues or others. As such, many methods have been developed to prevent these errors from interfering with user and developer experience. In React, one such method is the use of error boundaries.
Front-end developers often overlook error handling and logging. However, if any code segment throws an error, you must handle it correctly. Besides, depending on the situation, there are several ways to manage and log errors in React.
So, Error boundaries are React components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of the component tree that crashed. Error boundaries catch errors during rendering, in lifecycle methods, and in constructors of the whole tree below them.
The react-error-boundary library greatly simplifies error handling in React and is the most effective solution to overcome the limitations of the basic error boundaries.
It enables you to display a fallback component, log errors just like in the basic error boundaries, and reset the application’s state, so the error does not occur again.
Installation
This module is distributed via npm which is bundled with node and should be installed as one of your project’s dependencies
:npm install –save react-error-boundary
The simplest way to use <ErrorBoundary>
is to wrap it around any component that may throw an error. This will handle errors thrown by that component and its descendants too.
Just like in the below code. I have wrapped ErrorBoundary to my main routing and provided FallbackComponent as my ErrorPage(for which code is being written below). So whenever my application will encounter any error which break our code or any api related issues(like CORS)..ErrorBoundary will handle it and will render ErrorPage.import { ErrorBoundary } from ‘react-error-boundary’;
[React]
FallBack Component: This is a component which will rendered in the event of an error. As props it will be passed the error
and resetErrorBoundary
(which will reset the error boundary’s state when called, useful for a “try again” button).
In Our code, resetErrorBoundary
is resetting the error boundary’s state on clicking “Go To Home Screen” button.
We can also react to errors (e.g. for logging) by providing an onError
callback:
So, you can use react-error-boundary to eliminate the need for two different error handling methods in your app
Limitation
Error boundaries do not catch errors for:
- Event handlers
- Asynchronous code (e.g.
setTimeout
orrequestAnimationFrame
callbacks) - Server side rendering
- Errors thrown in the error boundary itself (rather than its children)
Conclusion
Any high-quality application must handle errors and unexpected events. Errors should be handled and appropriately logged to assist you in determining the root cause of errors while having the least impact on the user experience.
This article discussed various approaches that could be used to accomplish this, and I hope it will help you develop more robust React applications.