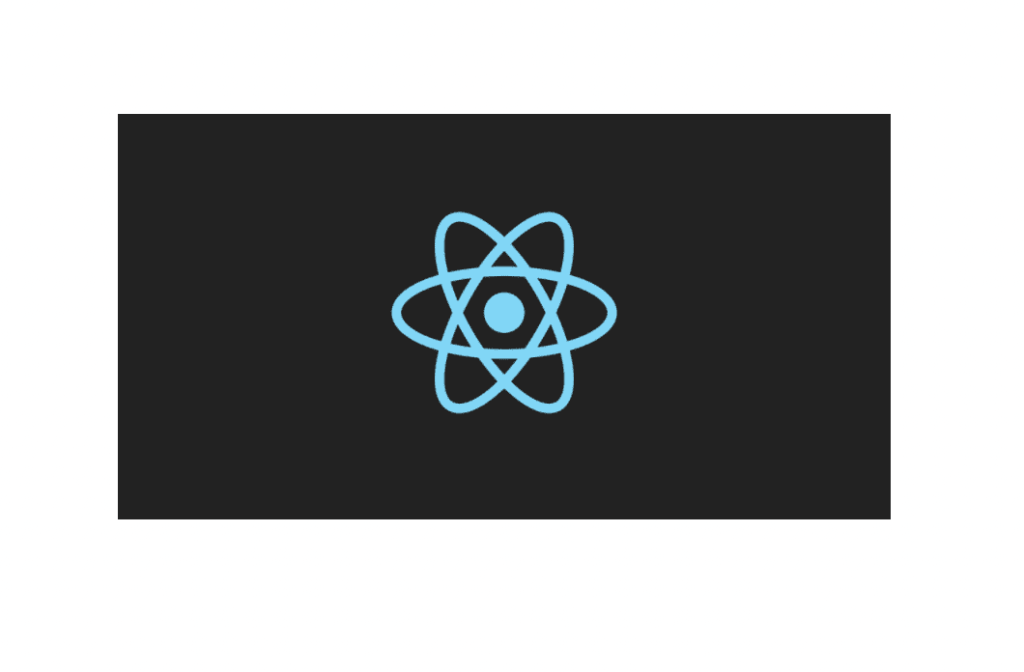
The title of the article might indicate that it’s going to talk about a way, a technique to make useMemo more efficient. However, this article intends to memoize the useMemo hook of react in your mind.
useMemo is one of the ways React provides to stop the unnecessary calls for expensive or time taking computations making the application fast and efficient.
[React] const Value= useMemo((params)=>{ function },[dependency list]) [React]//Value: constant holding value of the function.
//params: parameters(if any) required by the code.
//function: function that is to be memoized.
//dependency list: list of inputs on whose modification the function is re-run.
The above stated is an outline of memoisation of a function using useMemo. The memoized function is only executed when one or more inputs in the dependency list change. If the inputs remain same, an already cached/memoized value of the function is returned, without running the function, thus saving us time.
Let’s dive into code:
Let’s suppose we are displaying result of 2 computations in our component, and a button to perform computation for each component. Assuming that one component is light and the other one is heavy.
http://sh017.hostgator.tempwebhost.net/media/8dbfb33587c4cf2002611565dc514754
We can see that even while performing light computation, there is an unexpected delay. This happens due to the fact that, whenever we change the state, the component re renders and as the heavy computation is performed every time the component renders, there is a delay even when the button for performing light computation is pressed.
Let’s now see the same functionality with the magic of useMemo.
http://sh017.hostgator.tempwebhost.net/media/8dbfb33587c4cf2002611565dc514754
By using useMemo, it’s checked that whether the state updated affects the heavy computation or not. If not, a previously cached value of the computation is returned. Hence, the delay for all the computations other than the heavy one is eradicated.
Since it makes our app so efficient, one might be lured to use useMemo hook everywhere in the application. It would optimize the performance, wouldn’t it? Sadly, it’s not so. The useMemo hook has some performance and some memory overhead too. The useMemo hook is called every time the component renders (performance overhead) and it saves the cached value of the function (memory overhead), hence increasing the memory consumption by the application. Thus, it could cause performance problems if used in a rampant way.
Also, as we are executing a function/computation only on change of certain state/states, it’s natural to think of using the useEffect hook instead. The use cases of useEffect hook are quite similar, however there is a minor difference between useEffect and useMemo. The useEffect ensures that a function is called only on change of certain states but, the value computed by the function placed inside the useEffect can not be stored and assigned. This is where the useMemo hook comes to our rescue.
[React] useEffect((params)=>{ function },[dependency array]) const value=useMemo((params)=>{ function },[dependency array]); [React]Above attached is a code block with basic outline of both useEffect and useMemo hook, with which we come to the end of this article. I hope the usage and functioning of the useMemo hook is clear, and that you have a memoized version of useMemo with yourself.