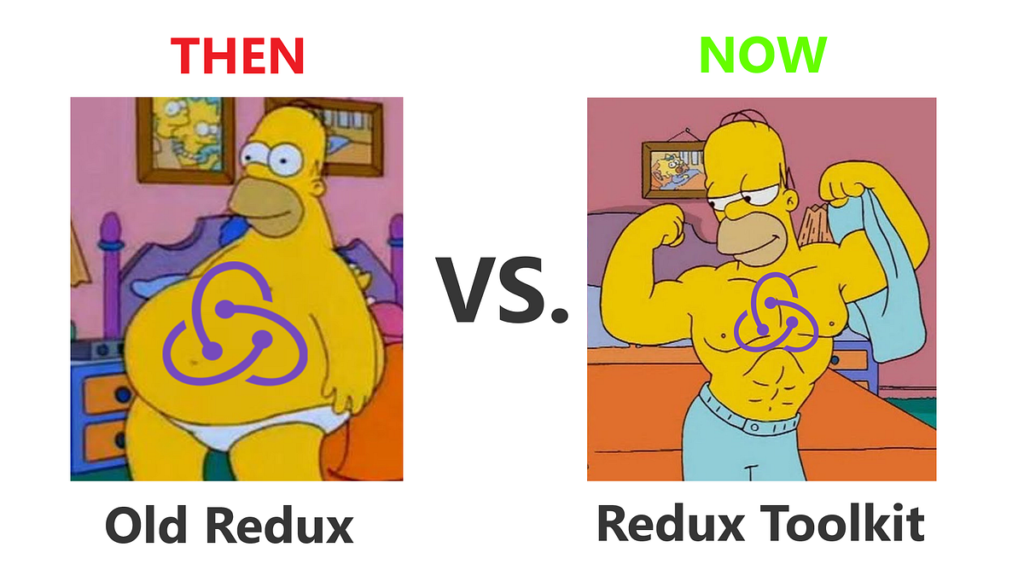
Before getting into this article, I’m assuming that you must be aware of redux basics, but for the sake of those who aren’t aware let’s look into it briefly.
Redux
Redux is a state management library that you can use with any JS library or framework like React, Angular, or Vue. It is one of the hottest libraries in front-end development these days. The documentation states that Redux is a predictable state container for JavaScript apps. In simple words, it’s an application data-flow architecture, rather than a traditional library or a framework like Underscore.js and AngularJS.
Data Flow

Its core building blocks such as a store, actions, and reducers, all come together and make Redux the global state management library.
A state is a read-only object that holds the application data that is shared between components. The only way to change the state is to dispatch an action. An action is an event that describes something that happened in the application.
A reducer is a function that receives the current state
and an action
object decides how to update the state if necessary, and returns the new state: (state, action) => newState.
The current Redux application state lives in an object called the store.
You can know redux in detail from here.
Now, let’s start with Redux Toolkit and see how it is smarter than Redux.
Redux Toolkit is a SOPE library that stands for Simple, Opinionated, Powerful, and Effective state management library. It allows us to write more efficient code, speed up the development process, and automatically apply the best-recommended practices.
Setup
# NPM npm install @reduxjs/toolkit //or # Yarn yarn add @reduxjs/toolkitIf you want to add Redux Toolkit and React-Redux packages :
npm install @reduxjs/toolkit react-reduxRTK was mainly created to solve the three major issues with Redux:
- Configuring a Redux store is too complicated
- Have to add a lot of packages to build a large-scale application
- Redux requires too much boilerplate code which makes it difficult to write efficient and clean code.
To understand the concept of the redux toolkit easily let’s see an example of Counter App
[typescript] //1. Create a Redux Store //store.ts import { configureStore } from ‘@reduxjs/toolkit’; import { counterReducer } from ‘./Reducer’; export const store = configureStore({ reducer: { counter: counterReducer, }, }); export type RootState = ReturnTypeRedux Toolkit comes with dependencies like immer, redux, redux-thunk, and reselect. It also provides automatic support for Redux Dev-tools Extension and for the immer.js library which is a great tool to deal with immutable objects.
From the above example, a basic comparison between redux and redux-toolkit:
Modifying The State:
//1. Redux: Need to handle manually and change the state immutably. const initialState={counter:0} const handleCounter=(state=initialState,action){ return{ …state, counter: state.counter+1; } } //2. Redux Toolkit: Provides the support for immer.js library which automatically changes the code immutably. const initialState={counter:0} const handleCounter=(state=initialState,action){ return{ counter: state.counter+1; } }Creating Store and Reducers.
//1. Redux const incrementHandler=(state=0,action)=>{ if(action.type===’INCREMENT’) { return state+1; } return state; } const store = createStore(incrementHandler); store.dispatch({type:’INCREMENT’}); //2. Redux Toolkit // Action Creators const increment= createAction(‘INCREMENT’); const incrementHandler = createReducer(0, { [increment]: state => state + 1 }) const store = configureStore({ reducer: incrementHandler }) store.dispatch(increment());Some functions or utilities provided by RTK:
createAction(): Returns an action creator function.
createSlice(): Replace create action and create Reducer functions with a single function.
configureStore(): Provides automatic support for Redux-thunk, Redux DevTools Extension, and also passes the default middleware.
createReducer(): Provides support for the immer library that allows writing the immutable code mutably.
As you can see, the Redux Toolkit code is simpler and requires less boilerplate code than the Redux code. The various predefined functions speed up the process and save the time required to manage the state of an application.
If you want to know more about Redux Toolkit, please check here.