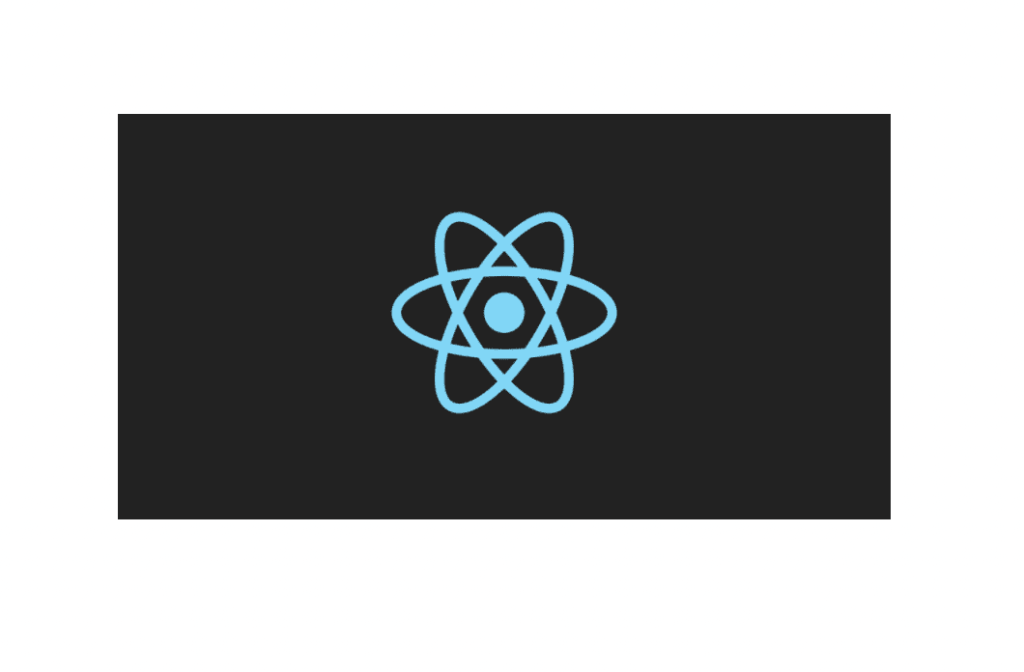
In React, forwardRef and useImperativeHandle are two features that are used to communicate between child and parent components. forwardRef allows a component to pass a ref to its child component, and useImperativeHandle allows a child component to expose some of its functions to its parent. In this article, we’ll look at how to use these two features in React.
Using forwardRef
In React, forwardRef is a Higher-Order Component (HOC) that allows a component to pass a ref to its child component. It’s commonly used to access child components’ functions or properties that are not available via props. To use forwardRef, you need to create a function that accepts two arguments: props and ref. Then, pass ref to the child component.
Create a new component
Input.jsx
[React] import { forwardRef } from “react”; const Input = forwardRef((props, ref) => { return ; }); export default Input; [React]App.jsx
[React] const App = () => { const inputRef = React.useRef(); const handleInputFocus = () => { inputRef.current?.focus(); }; return (In this example, we have created an Input component that accepts a ref and returns an input element with that ref. In the parent component (App), we created a ref for the Input component and passed it to the ref prop. Then, we created a button that calls the focus method of the inputRef when clicked.
Using useImperativeHandle
useImperativeHandle is a hook that allows a child component to expose some functions to the parent component. It’s used when you want to call a function that’s inside the child component from the parent component. To use useImperativeHandle, you need to create a ref and pass it to the child component.
Here’s an example:
[React] import React, { forwardRef, useImperativeHandle, useRef } from “react”; const Input = forwardRef((props, ref) => { const inputRef = useRef(); useImperativeHandle(ref, () => { const focusInput = () => { inputRef.current?.focus(); }; return { focusInput }; }); return ; }); export default Input; [React]And now we can use the new component like this
[React] const App = () => { const inputRef = React.useRef(); const handleInputFocus = () => { inputRef.current?.focusInput(); }; return (In this example, we created a Child component that accepts a ref and returns an input element with that ref. We also created a useImperativeHandle hook that exposes the focusInput function to its parent component. In the parent component (App), we created a ref for the Child component and passed it to the ref prop. Then, we created a button that calls the focusInput method of the childRef when clicked.
Conclusion
In this article, we looked at how to use forwardRef and useImperativeHandle in React to communicate between child and parent components. These two features are essential when you want to access child from the parent.