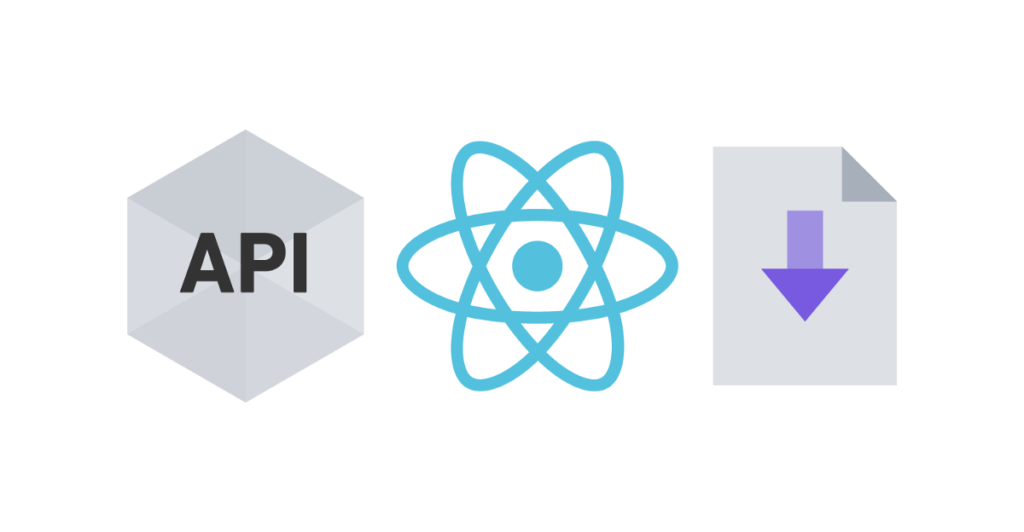
RNFetchBlob is a library, which is used for file downloading and uploading. It is committed to making file access and transfer more accessible and efficient for React Native developers. We’ve implemented a highly customizable filesystem and network module which plays well together. For example, developers can upload and download data directly from/to storage, which is more efficient, especially for large files. The file will be downloaded to DownloadDir on Android and DocumentDir on iOS depending on the current platform.
Features:
- File API supports regular files, Asset files, and CameraRoll files.
- Native-to-native file manipulation API, reduces JS bridging performance loss.
- File stream support for dealing with large file
- Blob, File, XMLHttpRequest polyfills that make browser-based libraries available in RN.
- Transferring the data directly from/to storage without BASE64 bridging
Installation of Package:
Using npm –
install –save rn-fetch-blob
Using yarn –
yarn add rn-fetch-blob
Now, there’s some additional configuration that needs to be done in order to download files with RNFetchBlob.
Since we will use Android Download Manager, granting permission to external storage for android, we have to add the following piece of code on AndroidManifest.xml
Note:
If you also plan on restricting the download to wifi, then you’ll also need the following permission in the manifest file.
I am taking example for download invoice from remote, so let’s following the code :
import RNFetchBlob from 'rn-fetch-blob'; const actualDownload = () => { const { dirs } = RNFetchBlob.fs; const dirToSave = Platform.OS === 'ios' ? dirs.DocumentDir : dirs.DownloadDir; const configfb = { fileCache: true, addAndroidDownloads: { useDownloadManager: true, notification: true, mediaScannable: true, title: `Invoice.pdf`, path: `${dirs.DownloadDir}/Invoice.pdf`, }, useDownloadManager: true, notification: true, mediaScannable: true, title: 'Invoice.pdf', path: `${dirToSave}/Invoice.pdf`, }; const configOptions = Platform.select({ ios: configfb, android: configfb, }); RNFetchBlob.config(configOptions || {}) .fetch('GET', invoiceUrl, {}) .then(res => { if (Platform.OS === 'ios') { RNFetchBlob.fs.writeFile(configfb.path, res.data, 'base64'); RNFetchBlob.ios.previewDocument(configfb.path); } if (Platform.OS === 'android') { console.log("file downloaded") } }) .catch(e => { console.log('invoice Download==>', e); }); };const getPermission = async () => { if (Platform.OS === 'ios') { actualDownload(); } else { try { const granted = await PermissionsAndroid.request( PermissionsAndroid.PERMISSIONS.WRITE_EXTERNAL_STORAGE, ); if (granted === PermissionsAndroid.RESULTS.GRANTED) { actualDownload(); } else { console.log("please grant permission"); } } catch (err) { console.log("display error",err) } } };
Then call the getPermission() as for your required action.
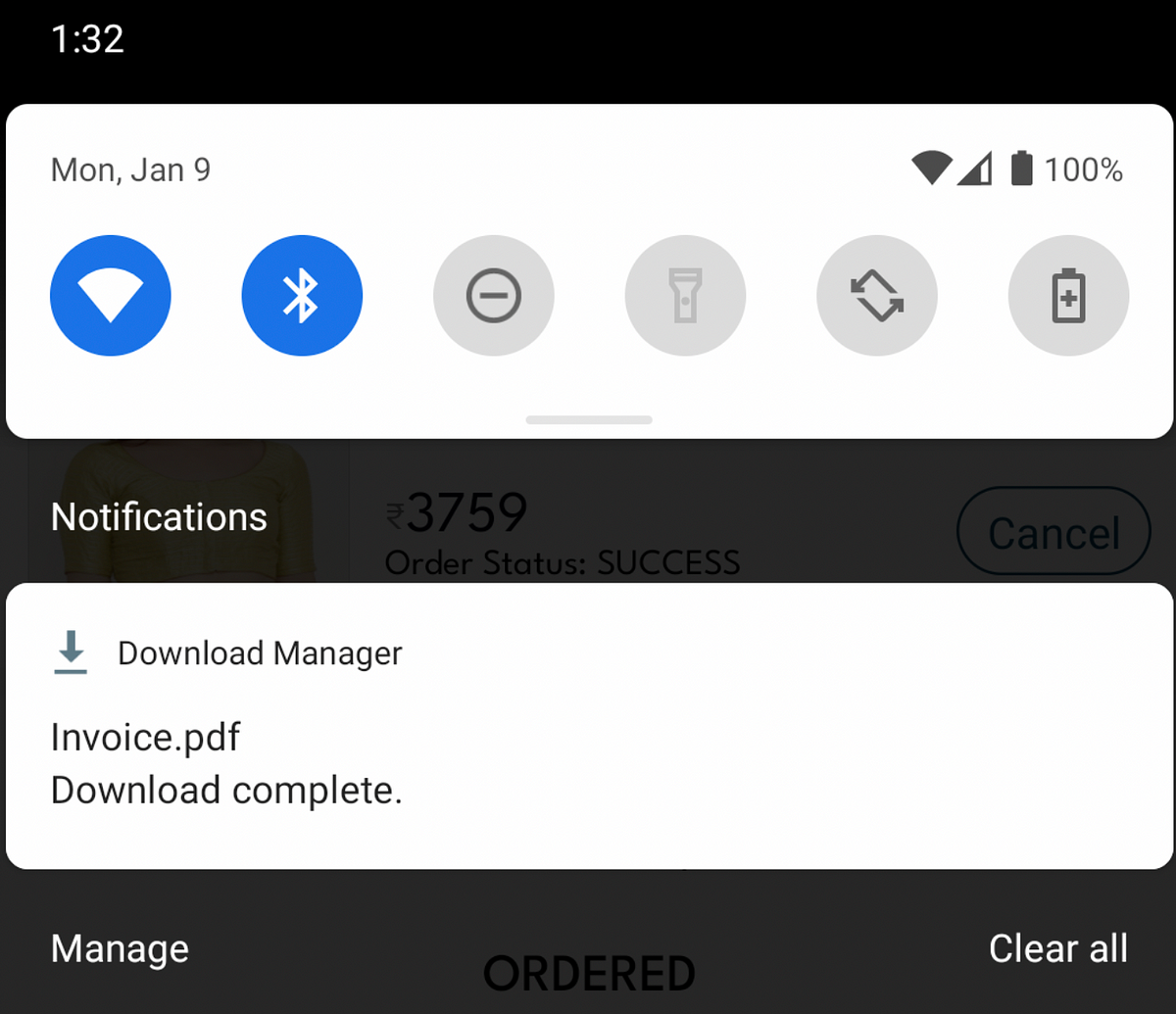