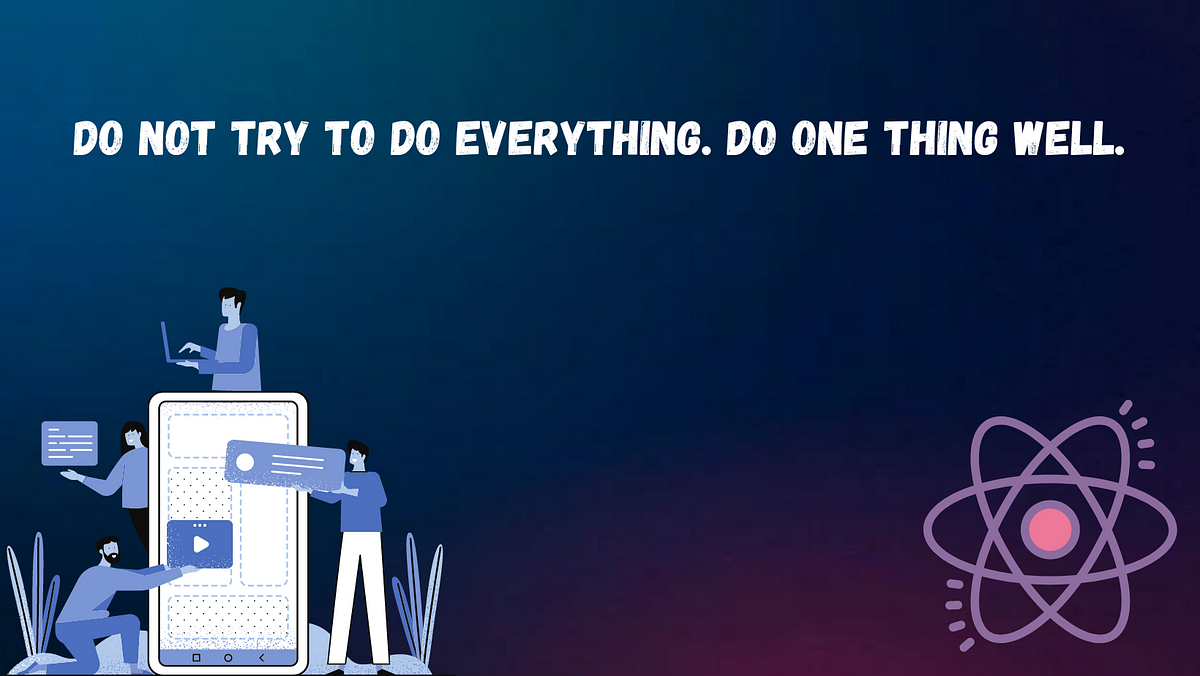
Imagine you are building a toy car out of Legos. The toy car has different parts, like wheels, a body, and a steering wheel. Each of these parts is like a piece of a puzzle, and when you put them all together, you get a complete toy car.
In React, each piece of the toy car (or each part of the puzzle) is called a “component”. Just like how the toy car has different parts that you can build and put together, a React app has different components that you can build and put together to create something bigger.
Now, imagine that you have already built your toy car and you are playing with it. Suddenly, you decide that you want to change the colour of the steering wheel from blue to red. In order to do this, you must take apart the toy car, replace the steering wheel with a red one, and then put the toy car back together again.
In React, when you change something about a component, the whole component has to be “re-rendered”, or rebuilt. This is like taking apart the toy car and putting it back together again. Sometimes, re-rendering a component is necessary and it’s not a big deal. But other times, re-rendering a component can be a waste of time and resources, significantly if you are only changing a small part of the component.
That’s where “pure components” come in. A pure component is like a toy car that has a special way of building itself. Instead of rebuilding the whole toy car every time you want to change something, a pure component will only rebuild the parts that need to be changed. This is more efficient and can make your React app run faster.
Technically, a “pure component” is defined as a component that renders the same output for the same state and props by internally implementing
shouldComponentUpdate
but with a shallow prop and state comparison.
How to create a pure class-based component?
Pure Components in Class Components can be used using React.PureComponent and implemented as follow:
import React from 'react'; class MyComponent extends React.PureComponent { render() { // render component using this.props } }
How to create a pure functional-based component?
Pure Components in function-based components can be implemented using React.memo.
REACT.MEMO
IS A HIGHER ORDER COMPONENT.If your component renders the same result given the same props, you can wrap it in a call to
React.memo
for a performance boost in some cases by memoizing the result. This means that React will skip rendering the component, and reuse the last rendered result.
import React from 'react'; const MyComponent = React.memo(function MyComponent(props) { // render component using props });
Conclusion
Since we have completely moved to function-based components with the React.memo()
API, you can enjoy the performance benefits that come from using functional components together with optimizations that come with memoizing the components.
Happy Coding!!🥂 🎉